문제
(세 자리 수) × (세 자리 수)는 다음과 같은 과정을 통하여 이루어진다.
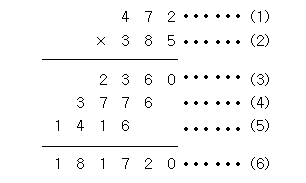
(1)과 (2)위치에 들어갈 세 자리 자연수가 주어질 때 (3), (4), (5), (6)위치에 들어갈 값을 구하는 프로그램을 작성하시오.
입력
첫째 줄에 (1)의 위치에 들어갈 세 자리 자연수가, 둘째 줄에 (2)의 위치에 들어갈 세자리 자연수가 주어진다.
출력
첫째 줄부터 넷째 줄까지 차례대로 (3), (4), (5), (6)에 들어갈 값을 출력한다.
풀이 과정
(3) - (1)과 (2)의 일의 자리 수를 곱한 값
(4) - (1)과 (2)의 십의 자리 수를 곱한 값
(5) - (1)과 (2)의 백의 자리 수를 곱한 값
(6) - (1)과 (2)를 곱한 값
이를 차례대로 출력한다.
C
#include <stdio.h>
int main(void) {
int a, b;
scanf("%d %d", &a, &b);
printf("%d\n", a*(b%10));
printf("%d\n", a*((b%100)/10));
printf("%d\n", a*(b/100));
printf("%d\n", a*b);
return 0;
}
C++
#include <iostream>
using namespace std;
int main(void) {
int a, b; cin >> a >> b;
cout << a*(b%10) << endl << a*((b%100)/10) << endl << a*(b/100) << endl << a*b;
return 0;
}
Python
a = int(input())
b = int(input())
print(a*(b%10))
print(a*((b%100)//10))
print(a*(b//100))
print(a*b)
Java
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int a = sc.nextInt();
int b = sc.nextInt();
System.out.println(a*(b%10));
System.out.println(a*((b%100)/10));
System.out.println(a*(b/100));
System.out.println(a*b);
}
}
'-- 예전 기록 > BOJ' 카테고리의 다른 글
[ BOJ ] 11382 : 꼬마 정민 ( BRONZE 5 ) / C, C++, Python, Java (0) | 2023.07.04 |
---|---|
[ BOJ ] 13909 : 창문 닫기 ( SILVER 5 ) / Python (0) | 2023.07.03 |
[ BOJ ] 16139 : 인간-컴퓨터 상호작용 ( SILVER 1 ) / Python (0) | 2023.06.30 |
[ BOJ ] 10430 : 나머지 ( BRONZE 5 ) / C, C++, Python, Java (0) | 2023.06.30 |
[ BOJ ] 2485 : 가로수 ( SILVER 4 ) / Python (0) | 2023.06.26 |